티스토리 뷰
[Android] 안드로이드 - Text 입력 이벤트 처리 - TextWatcher
Lkt_Programmer 2019. 6. 23. 13:54안드로이드 앱에서 사용자로부터 값을 입력받을 때 사용되는 View 위젯 중 EditText가 있습니다. 앱을 구현하다 보면 이러한 EditText의 Text 입력값이 변경될 때마다 특정 작업을 처리해야 할 경우가 있습니다.
이때는 TextWatcher 인터페이스를 구현하여 EditText의 이벤트 리스너로 등록하여 처리가 가능합니다.
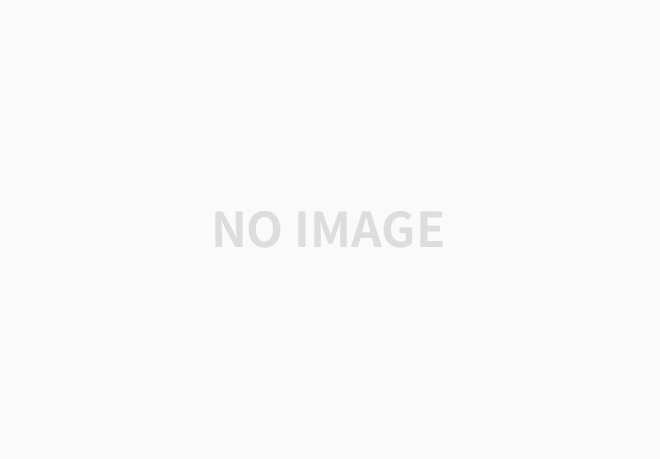
1. TextWatcher 인터페이스 필구 구현 함수들
■ beforeTextChanged(CharSequence s. int start, int count, int after)
-
CharSequece s : 현재 EditText에 입력된 값
-
int start : s에 저장된 문자열에서 새로 추가될 문자열의 시작 위치 값
-
int count : s에 새로운 문자열이 추가된 후 문자열의 길이
-
int after : 새로 추가될 문자열의 길이
■ onTextChanger(CharSequence s, int start, int before, int count)
start 위치에서 before 문자열 개수만큼 문자열이 count 개수만큼 변경되었을 때 호출
-
CharSequence s : 새로 입력한 문자열이 추가된 EditText의 값을 가지고 있음
-
int start : 새로 추가된 문자열의 시작 위치 값
-
int before : 삭제된 기존 문자열의 개수
-
int count : 새로 추가된 문자열의 개수
■ afterTextChanged(Editalbe a)
EditText의 Text가 변경된 것을 다른 곳에 통보할 때 사용됩니다. a.toString()을 통해 현재 EditText의 Text 값을 불러오는 게 가능합니다. EditText의 Text 변경에 따른 함수 호출 순서는 beforeTextChanged, onTextChanger, afterTextChanged 순으로 호출됩니다.
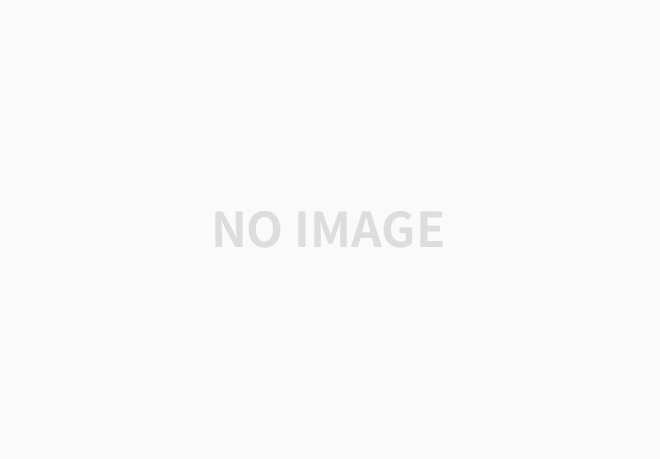
2. TextWatcher 구현 예제
먼저 Main 화면의 Xml 레이아웃 리소스입니다.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:text=""
android:textSize="50dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@id/editText" />
</android.support.constraint.ConstraintLayout>
▼ EditText 하나와 TextView 하나를 배치한 형태입니다. TextWatcher 구현체를 등록한 EdiText의 값이 변경될 때마다 TextView의 Text에 반영되도록 구현할 것입니다.
package com.springsthursday.textwatcher;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.getViewObject();
editText.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
}
@Override
public void afterTextChanged(Editable s) {
textView.setText(s.toString());
}
});
}
private void getViewObject()
{
editText = findViewById(R.id.editText);
textView = findViewById(R.id.textView);
}
}
▼ getViewObject() 함수에서 레이아웃 리소스 상의 TextView와 EditText의 참조 객체를 얻어옵니다. onCreate() 함수에서는 EditText의 addTextChangedListener() 함수를 통해 Text 값 변경에 따른 이벤트 리스너를 등록해주는데 익명 클래스 작성법을 통하여 TextWatcher() 인터페이스를 구현한 객체를 전달합니다.
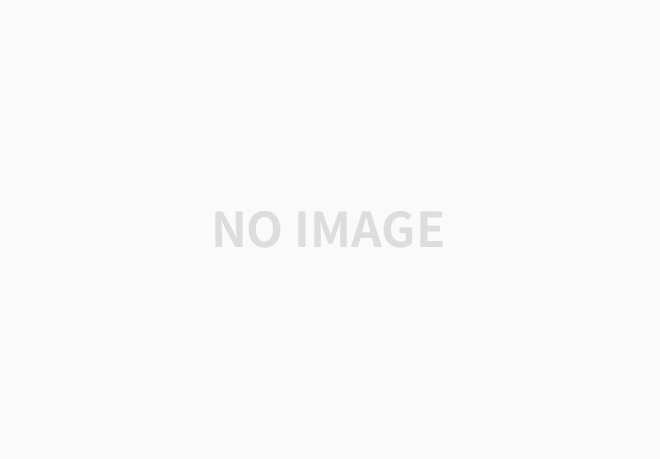
3. 참조
■ TextWatcher 구현 관련 안드로이드 공식 참조 문서
https://developer.android.com/reference/android/text/TextWatcher
■ 익명 클래스 사용법 / 이벤트 리스너 구현 방식
[Android] 안드로이드 - 익명 클래스(Anonymous Class) 사용법
[Android] 안드로이드 - 버튼 이벤트 처리방법 정리 (리스너 구현 및 이벤트 핸들링)
'Programming > Android 개발' 카테고리의 다른 글
[Android] 안드로이드 - 갤러리에서 이미지 가져오기 (3) | 2019.07.04 |
---|---|
[Android] 안드로이드 - 플로팅 액션 버튼(Floating Action Button) 사용법 (0) | 2019.06.24 |
[Android] 안드로이드 - KeyEvent(키 이벤트) 처리 (1) | 2019.06.22 |
[Android] 안드로이드 - BottomNavigationView 사용하여 하단 메뉴 만들기 (1) | 2019.06.21 |
[Android] 안드로이드 - 프래그먼트 (Fragment) 사용하기 (1) | 2019.06.20 |